How to Send Transactions on Ethereum
This is a beginner's guide for sending Ethereum transactions in web3.
There are three main steps in order to send a transaction to the Ethereum blockchain: create, sign, and broadcast. We'll go through all three, hopefully answering any questions you might have! In this tutorial, we'll be using Alchemy to send our transactions to the Ethereum chain. You can create a free Alchemy account here.
Note
This guide is for signing your transactions on the backend for your app, if you want to integrate signing your transactions on the frontend, you'll need to integrate a browser provider with Web3.
The Basics
Like most blockchain developers when they first start, you might have done some research on how to send a transaction (something that should be pretty simple) and ran into a plethora of guides, each saying different things and leaving you a bit overwhelmed and confused. If you're in that boat, don't worry; we all were at some point! So, before we start, let's get a few things straight:
1. Alchemy does not store your private keys
- This means that Alchemy's servers cannot sign and send transactions on your behalf. The reason for this is security purposes. Alchemy will never ask you to share your private key, and you should never share your private key with a hosted node (or anyone for that matter).
- However, you can use the Wallet in the Alchemy SDK to sign your transactions. This Wallet exists only on your machine running the code, and cannot share your private key with anyone else.
- You can read from the blockchain using Alchemy's core API, but to write to it you'll need to use the Alchemy SDK or an external wallet to sign your transactions before sending them through Alchemy.
2. What is a "signer"?
- Signers will sign transactions for you using your private key. In this tutorial, we'll be using the Alchemy SDK to sign our transaction, but you could also use any other web3 library. The Alchemy SDK Wallet implements a Signer.
- In the frontend, an excellent example of a signer would be Metamask, which will sign and send transactions on your behalf.
3. Why do I need to sign my transactions?
- Every user that wants to send a transaction on the Ethereum network must sign the transaction first in order to validate that the origin of the transaction is who it claims to be.
- It is super important to protect this private key, since having access to it grants full control over your Ethereum account, allowing you (or anyone with access) to perform transactions on your behalf.
4. How do I protect my private key?
- There are many ways to protect your private key and to use it to send off transactions. In this tutorial, we will be using a
.env
file. However, you could also use a separate provider that stores private keys, use a Keystore file, or other options.
5. What is the web3 library?
- The Alchemy SDK is a wrapper library around the standard JSON-RPC calls that are quite common to use in Ethereum development.
- There are many different web3 libraries for different languages. In this tutorial, we'll use the Alchemy SDK which is written in JavaScript.
Okay, now that we have a few of these questions out of the way, let's move on to the tutorial. Feel free to ask questions anytime in our discord!
Note
This guide assumes you have an Alchemy account, an Ethereum address or Metamask wallet, Node.js, and npm installed. If not, follow these steps:
- Create a free Alchemy account
- Create a Metamask account (or get an Ethereum account)
- Follow these steps to install NodeJs and NPM
Steps to Sending Your Transaction
1. Create an Alchemy app on the Sepolia testnet
Navigate to your Alchemy Dashboard and create a new app, choosing Sepolia for your network. (In practice, you could use any testnet of your choice, but for this guide, we're sticking to Sepolia.)
Choosing a testnet
While you can use the Goerli testnet, we caution against it as the Ethereum Foundation has announced that Goerli will soon be deprecated.
We therefore recommend using Sepolia as Alchemy has full Sepolia support and a free Sepolia faucet also.
2. Request Eth from the Alchemy Sepolia faucet
Follow the instructions on the faucet homepage to receive Eth. Make sure to include your Sepolia Ethereum address (from Metamask) and not another network. After following the instructions, double-check that you've received the Eth in your wallet.
3. Create a new project directory and cd
into it
cd
into itCreate a new project directory from the command line (terminal for macs) and navigate into it:
mkdir sendtx-example
cd sendtx-example
4. Install the Alchemy SDK and dotenv
Run the following command in your project directory:
npm init --yes
npm install alchemy-sdk dotenv
5. Create the .env file
We'll use a .env
file to safely store our API key and private key.
.env
We make a .env file to securely store private environmental variables in our local machine that we may access from other files (some of which we can make public).
If you want to check out how
dotenv
actually works in the context of a conventional NodeJS server file, check out this helpful video!
Create a .env file (make sure the file is literally just named .env
, nothing more) in your project directory and add the following (replacing your-api-key
and your-private-key
, keeping both within the quotation marks):
- To find your Alchemy API Key, navigate to the app details page of the app you just created on your Alchemy dashboard, click "View Key" in the top right corner, and grab the Api Key.
- To find your private key using Metamask, check out this guide.
API_KEY = "your-api-key"
PRIVATE_KEY = "your-private-key"
6. Create sendTx.js
file
sendTx.js
fileGreat, now that we have our sensitive data protected in a .env
file, let's start coding. For our send transaction example, we'll be sending Eth back to the Sepolia faucet.
Create a sendTx.js
file, which is where we will configure and send our example transaction, and add the following lines of code to it:
const { Alchemy, Network, Wallet, Utils } = require("alchemy-sdk");
const dotenv = require("dotenv");
dotenv.config();
const { API_KEY, PRIVATE_KEY } = process.env;
const settings = {
apiKey: API_KEY,
network: Network.ETH_SEPOLIA,
};
const alchemy = new Alchemy(settings);
let wallet = new Wallet(PRIVATE_KEY);
async function main() {
const nonce = await alchemy.core.getTransactionCount(
wallet.address,
"latest"
);
let transaction = {
to: "0xa238b6008Bc2FBd9E386A5d4784511980cE504Cd",
value: Utils.parseEther("0.001"),
gasLimit: "21000",
maxPriorityFeePerGas: Utils.parseUnits("5", "gwei"),
maxFeePerGas: Utils.parseUnits("20", "gwei"),
nonce: nonce,
type: 2,
chainId: 11155111,
};
let rawTransaction = await wallet.signTransaction(transaction);
let tx = await alchemy.core.sendTransaction(rawTransaction);
console.log("Sent transaction", tx);
}
main();
Now, before we jump into running this code, let's talk about some of the components here.
Wallet
: This object stores your private key, and can be accessed to sign a transaction with your private key or to return your public address.nonce
: The nonce specification is used to keep track of the number of transactions sent from your address. We need this for security purposes and to prevent replay attacks. To get the number of transactions sent from your address we use eth_getTransactionCounttransaction
: The transaction object has a few aspects we need to specifyto
: This is the address we want to send Eth to. In this case, we are sending Eth back to the Sepolia faucet we initially requested from.gasLimit
: This is the maximum amount of gas you are willing to consume on a transaction. Standard limit is 21000 units.value
: This is the amount we wish to send, specified in wei where 10^18 wei = 1 ETHmaxFeePerGas
: This is the total amount you are willing to pay per gas for the transaction to execute. Since EIP 1559, this field or themaxPriorityFeePerGas
field is required. Specified in wei, where 10^18 wei = 1 ETHnonce
: see above nonce definition. Nonce starts counting from zero.- [OPTIONAL]
data
: Used for sending additional information with your transfer, or calling a smart contract, not required for balance transfers, check out the note below.
rawTransaction
: To sign our transaction object we will use thesignTransaction
method of our wallet, generating a raw "signed" transaction.sendTransaction
: Once we have a signed transaction, we can send it off to be included in a subsequent block by usingsendTransaction
A Note on
data
There are two main types of transactions that can be sent in Ethereum.
- Balance transfer: Send eth from one address to another. No
data
field is required, however, if you'd like to send additional information alongside your transaction, you can include that information in HEX format in this field.
- For example, let's say we wanted to write the hash of an IPFS document to the Ethereum chain in order to give it an immutable timestamp. Our data field should then look like
data: toHex(‘IPFS hash‘)
. And now anyone can query the chain and see when that document was added.- Smart contact transaction: Execute some smart contract code on the chain. In this case, the
data
field should contain the smart function you wish to execute, alongside any parameters.
- For a practical example, check out Step 6 in this tutorial: Interacting with a smart contract
7. Run the code using node sendTx.js
node sendTx.js
Navigate back to your terminal or command line and run:
node sendTx.js
8. See your transaction in the Mempool
Open up the Mempool page in your Alchemy dashboard and filter by the app you created to find your transaction. This is where we can watch our transaction transition from pending state to mined state (if successful) or dropped state if unsuccessful. Make sure to keep it on "All" so that you capture "mined", "pending", and "dropped" transactions. You can also search for your transaction by looking for transactions sent to address 0x31b98d14007bdee637298086988a0bbd31184523
To view the details of your transaction once you've found it, select the tx hash, which should take you to a view that looks like this:
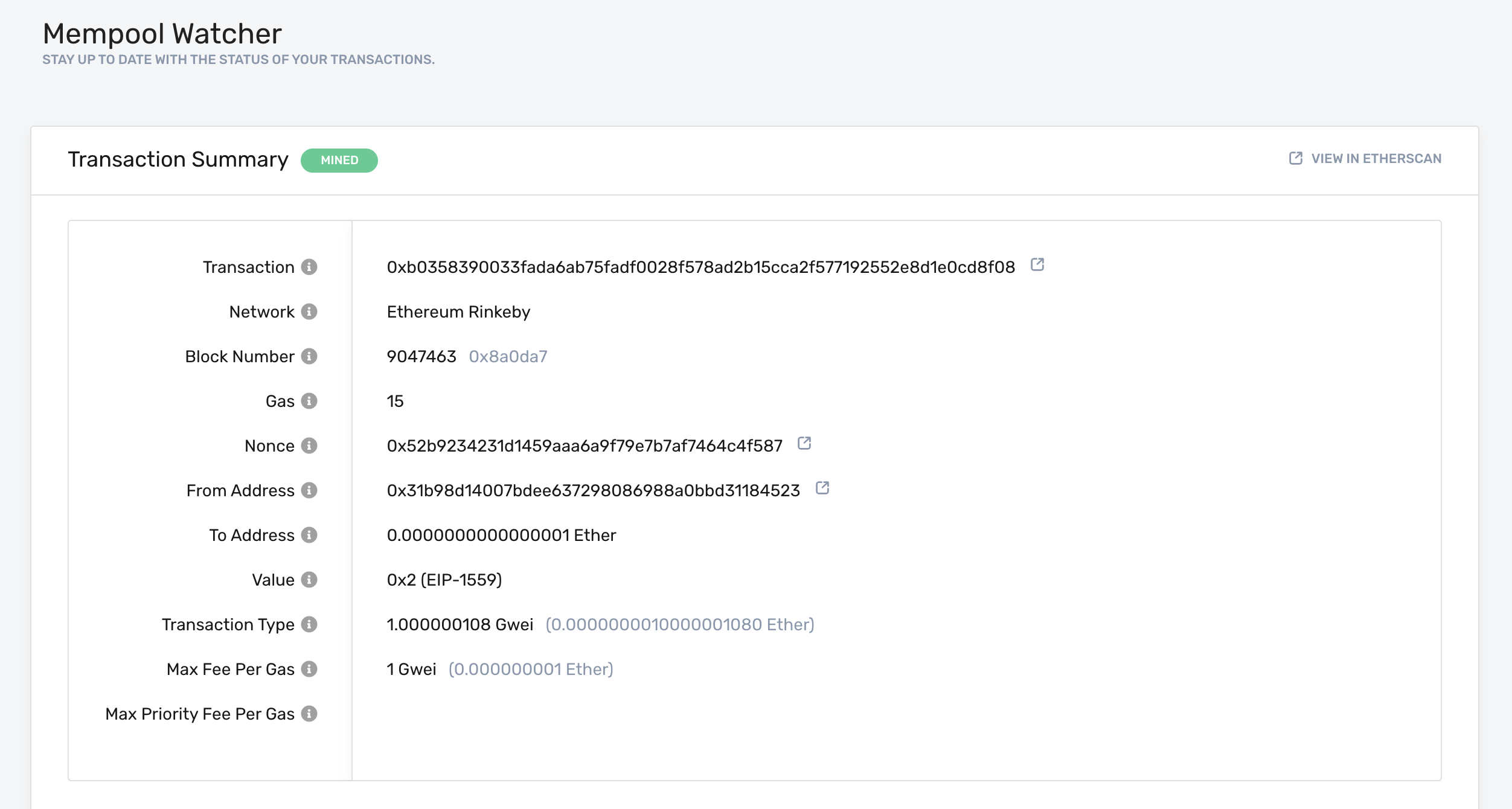
View your transaction on the Alchemy Mempool Watcher
From there you can view your transaction on Etherscan by clicking on the icon circled in red!
Yippieeee! You just sent your first Ethereum transaction using Alchemy 🎉
Once you complete this tutorial, let us know how your experience was or if you have any feedback by tagging us on Twitter @alchemyplatform!
_For feedback and suggestions about this guide, please message Deric on Alchemy's Discord! _
_Not sure what to do next? As a final test of your skills, get your hands dirty with some solidity programming by implementing our Hello World Smart Contract tutorial.
Updated almost 2 years ago