What are Solidity events?
Solidity events log information to the blockchain outside of smart contracts' storage variables using the event
keyword. They're emitted by smart contracts and read by connected code.
Previous Section Recap
In the previous section, we learned about [mappings](), one of the most used and important data structures in all of Solidity. A typical use case of a mapping would be to keep track of account balances by address:mapping(address => uint) public balances;
where address
is the key into the mapping and uint
is the value (the balance in this example).
Not only do we need this type of data in smart contracts all the time, but the fact that given an address, we can look its balance up in constant time O(1) time is a very important characteristic of mappings.
An excellent real-world example of mappings being used to map addresses to balances can be found in the ERC-20 token spec.
Events
Events are the way Solidity and the EVM provide developers with logging functionality used to write information to a data structure on the blockchain that lives outside of smart contracts' storage variables.
Events are an abstraction on top of the EVM's low-level logging functionality, opcodes LOG0
to LOG4
. The specific opcode used will depend on the number of topics the event declares using the indexed
keyword. A topic is just a variable that we want to be included in the event and tells Solidity we want to be able to filter on the variable as well.
The low-level logs are stored in the transaction receipt of the transaction under the transaction receipts trie. Logs are written by the smart contract when the contract emits events, but these logs cannot be ready by the smart contract. The inaccessability of the logs allows developers to store data on-chain that is more searchable and gas efficient than saving data to the smart contract's storage variables.
Defining Events
Events are defined in smart contracts using the event
keyword. Here is the transfer event from the ERC20 smart contract. It is emitted whenever tokens are transferred from 1 account to another.
interface IERC20 {
event Transfer(address indexed from, address indexed to, uint256 value);
}
Here we can see the different components of an event:
- the event's name
Transfer
- the event's topics
from
(sender's address),to
(the receiver's address),value
(the amount transferred) - if a variable in the event is not marked as
indexed
it will be included when the event is emitted, but code listening on the event will not be able to filter on non-indexed variables (aka topics).
Whenever a Transfer
event is emitted, the from
, to
and value
data will be contained in the event.
Emitting Events
Once an event has been defined we can emit the event from the smart contract. Continuing on from the ERC20 smart contract let's see where the Transfer
event is emitted.
function _transfer(
address from,
address to,
uint256 amount
) internal virtual {
// perform various checks, such as the `from` address has `amount` of tokens
// do the transfer of tokens
unchecked {
_balances[from] = fromBalance - amount;
_balances[to] += amount;
}
// the Transfer event is emitted here
emit Transfer(from, to, amount);
// perform various cleanup
}
Listening to Events
If you remember the definition of an Event from above, smart contracts can write events, but not read events. So how do we listen/read to data that smart contracts cannot read?
We listen to and read events from code connected to a provider. From what we've learned so far in this course we could do this in JS code using an ethers
provider to connect to a contract and listen to transfer events and do something with the event data.
const provider = new ethers.providers.Web3Provider(window.ethereum);
const contract = new Contract(erc20TokenAddress, ERC20_ABI, provider);
contract.on('Transfer', async (from, to, amount, data) => {
console.log('Transfer event emitted. {from, to, amount, data}');
});
Here we just simply print out the data of the event.
Finding Events in the Logs of a Transaction Receipt 🧾
In the above section we used the higher-level ethers
library to listen to Transfer
events and do something with them when they are emitted.
Going back to our lessons on Ethereum Nodes and the low-level JSON-RPC endpoints, we could also use eth_getLogs
to get at the same log data. The logs in the screenshot below were reading using Alchemy's Composer tool
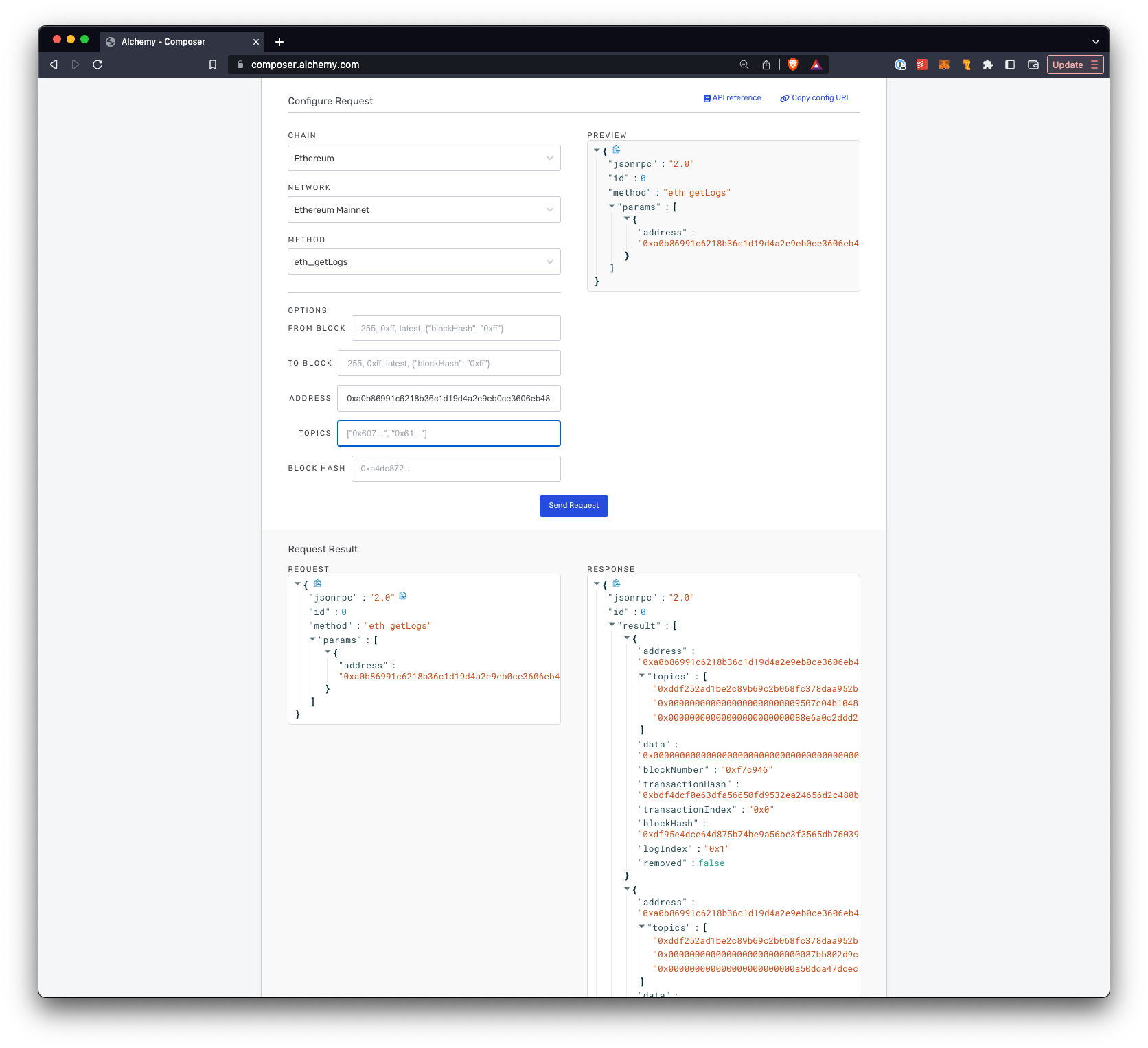
By using the lower level eth_getLogs
call you can see that we would need to write the code to loop through all the logs looking for the addresses, and values that we might specifically be interested in. A much less convenient way to do than to use higher-level library like ethers
.
Suggested Reading
- Solidity docs on Events and Indexed Topics
- More details about the LOG0, LOG1, LOG2 and LOG3 opcodes.
Questions ❓
Are events and logs part of the blockchain? What are your thoughts?
-
Events and logs are stored on the blockchain in transaction receipts
-
But they are not required for blockchain concensus
-
They are however verified by the blockchain since transaction receipt hashes are stored inside blocks
Can you think of any purpose of the LOG0 opcode?
LOG0 can be very useful for logging/debugging while building your contracts.
Conclusion
Events are a great way to emit information to the outside world of things happening with the blockchain. Emitted events can be found inside the Transaction Receipt of every transaction.
Learn More About Ethereum Development
Alchemy University offers free web3 development bootcamps that explain Solidity events and help developers master the fundamentals of web3 technology. Sign up for free, and start building today!
Updated over 1 year ago